IntelliTrace is one of the major new features in Visual Studio 2010. It’s a DVR on steroids for your debugging session. Unfortunately in it’s first iteration IntelliTrace was only available for 32 bit applications, which means SharePoint 2010 was a no-go. Turns out that with the Visual Studio 2010 Service Pack 1 beta, IntelliTrace is now available for x64 and, you guessed it, SharePoint 2010.
Apparently this is old news. The beta came out in December of last year, so forgive me for being a bit behind the times here. My December was pretty busy with SharePoint training, holiday shopping and last minute Microsoft certification preparation/exam, and I missed out on the announcement. But once I heard I was instantly excited. Can it really be true?
Sometimes when you want to try out something new and exciting, it turns out there is an excruciating setup and configuration period that just takes the wind right out of you. By the end, you just want to sit down and cry. To be honest, this was the experience I was expecting this to be, considering the complexity of SharePoint, IntelliTrace, and the fact that this is a beta release. That said, I don’t really know much about IntelliTrace. I saw it demoed a few times at last years TechEd, but I’ve never sat down and tried it myself. I just figured that something this cool must be really complicated.
Well I’m happy to tell you that it’s easy. I haven’t done anything crazy with it yet, but I jotted down the steps I did to get you up and going with using IntelliTrace with SharePoint 2010. Here’s what you need:
- SharePoint 2010
- Visual Studio 2010 Ultimate Edition
- Visual Studio 2010 Service Pack 1 beta
Before you step any further, keep in mind this is a beta release. Don’t install this on your development machine unless you snapshot it, or you’re OK with the potential of explosions. I’m not saying it’s a bad release, I haven’t had any issues, but there’s always a risk with betas.
I already had SharePoint 2010 installed as a single server farm, as well as Visual Studio 2010 Ultimate Edition (thanks Gary!). I downloaded the SP1 beta installer from the link above and got it going. It’s not very exciting, as installers usually aren’t. It also took quite a while.

Once I had done that, including a restart, I started up Visual Studio and made sure IntelliTrace was enabled. This may or may not be on by default. Mine was on, but I can’t recall whether I turned this on myself or if it was always like this. To turn it on, click Debug -> Options and Settings…

Make sure the checkbox that says “Enable IntelliTrace” in the “IntelliTrace” category is enabled. It’s pretty self-explainatory, but here’s a picture for good measure.

I also turned on IntelliTrace recordings, although this is optional. You can do this in the “Advanced” category” by checking the checkbox that says “Store IntelliTrace recordings in this directory:”.

That’s it. By this point I now had IntelliTrace enabled for use with SharePoint. No pain, no hassle. All puppies and rainbows. Obviously, just having it enabled is no good so I decided to take it for a spin and throw some easy code at it. I figured a Visual Web Part was probably an easy way to do some testing. I created one called FooBarWP and added a single button to it.

I double clicked the button and hooked up a simple throw Exception() to make the code explode when the button was pressed.
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
namespace IntelliTest.FooBarWP
{
public partial class FooBarWPUserControl : UserControl
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
throw new Exception("Something bombed");
}
}
}
Then I saved the Web Part and hit F5 to debug. After adding a page I added the newly created Web Part to said page before finally saving and closing editing mode.
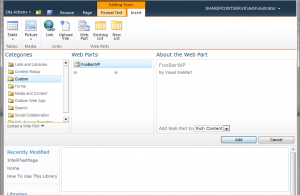
All was well so far. I hit the button, and obviously Visual Studio caught the exception like it always does. The difference from before was that IntelliTrace was now enabled. Which gave me this handy window:

There’s a lot of ASP.NET noise here, but you can enable/disable which events to listen to in the IntelliTrace settings. The neat thing is that you can click on events as they happened up the history, and get information from that point in the code (assuming there was code associated with the event). This even includes SQL calls to the database.
Going back to the Web Part, I added a TextBox to it and did some minor changes in the code.

using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Collections.Generic;
namespace IntelliTest.FooBarWP
{
public partial class FooBarWPUserControl : UserControl
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
List<string> stuff = new List<string>()
{
"Blah",
"Boo",
"Bee!"
};
Button1.Text = stuff[int.Parse(TextBox1.Text)];
}
}
}
This code is just a whole whackload of problems waiting to happen. There’s a parsing of the text value of a TextBox without making sure it’s a valid int32 and there’s an indexing into a List without making sure the index is within bounds. Bad code. Don’t ever put something like this in your software. Seriously.

Notice that near the top (8th from the top) of the IntelliTrace history, I’ve pressed the button with a 3 in the textbox. Later on I put in a 4 and pressed the button, which made it blow up. Like I mentioned before, you can also make it explode by entering something that isn’t a valid int32. Here’s what happened when I put in “six”:

This was right after the last exception, and you can see at the bottom of the history here, the button is named “Bee!”, which is what it changed to when I had 3 in the textbox and pressed the button. 6th from the top you can see there’s an event saying “Debugger: Stopped at Exception:”, which is the previous screenshot from above.
Not only can you catch exceptions like this, but you can also catch breakpoints. I set a normal break point on the same line of code, and this is what I got:
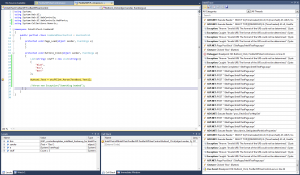
As you can see I’ve here hit the code before the exception occurs. I can see the history of the page and the actions taken. A very powerful way of troubleshooting for sure. These examples are all very simple, but it’s shows the basic setup and principle of using IntelliTrace together with SharePoint 2010.
Pretty cool stuff!
Leave a Reply